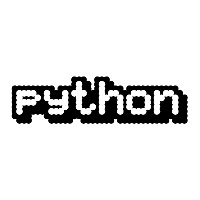
I have found that there is not a lot of documentation out there that shows you a simple way to instantiate a socket connection. Here is a simple code snippet, that shows you how to make a connection using the socket module. This would be useful for checking whether a host is listening on a particular port. This is not a full sockets implementation(no send, or recieve), but simply tests if a TCP socket is open.
import socket
#Simply change the host and port values
host = '127.0.0.1'
port = '80'
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
try:
s.connect((host, port))
s.shutdown(2)
print "Success connecting to "
print host + " on port: " + str(port)
except:
print "Cannot connect to "
print host + " on port: " + str(port)
FYI- You can test for a UDP port by changing "socket.SOCK_STREAM" to "socket.SOCK_DGRAM" .
Check out the documentation for the socket module here.
No comments:
Post a Comment